Before you begin, you need to have have both the Java Development Kit (JDK) and an application server installed on localhost. In this example I used Glassfish, which has an autodeploy directory. When an EJB jar file is dropped into the autodeploy directory, the application server automatically deploys it. To download the JDK, click here. To download Glassfish, click here.
Step 1: Create new EJB project
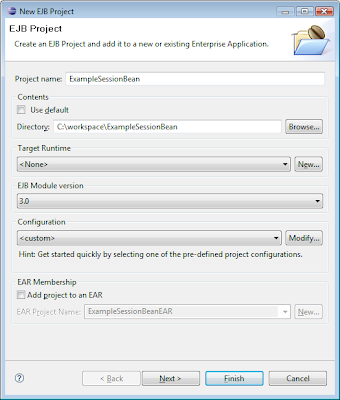
Let Eclipse generate the EJB-deployment descriptor.
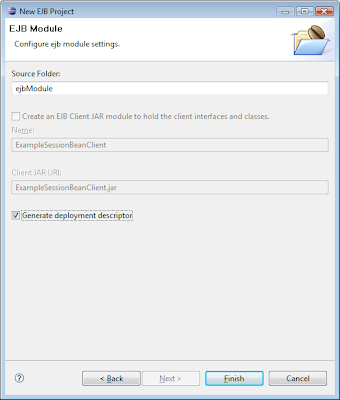
Include two Java EE jars (appserv-rt.jar and javaee.jar) which is needed to compile the beans. You can find the jars in the lib directory of your Glassfish installation directory. Add the jars to your project build path (Right-click on project, then click Build Path).
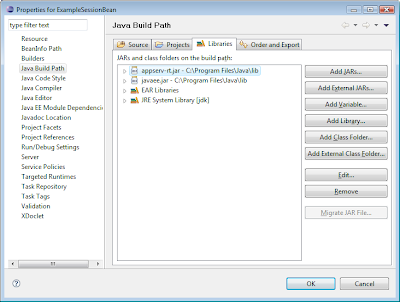
Step 3: Create the EJB Stateless Session Bean
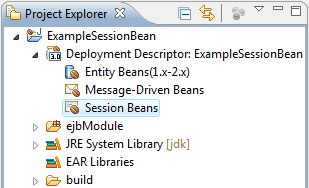
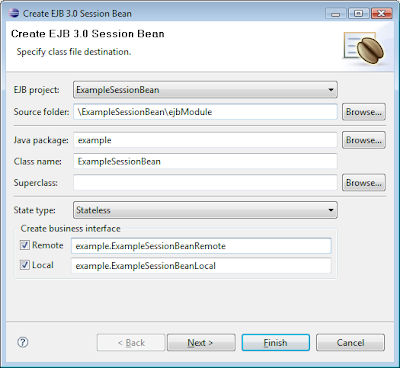
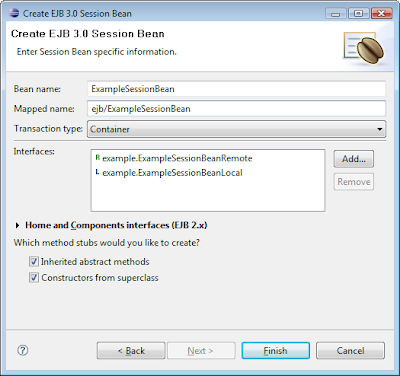
When you finish this step, you will find three skeleton class files of your bean. Make sure they look like the code examples below:
ExampleSessionBean source:
package example;
import javax.ejb.Stateless;
@Stateless(name = "ExampleSessionBean",
mappedName = "ejb/ExampleSessionBean")
public class ExampleSessionBean implements
ExampleSessionBeanRemote, ExampleSessionBeanLocal {
public ExampleSessionBean() {
;
}
public int multiply(int a, int b) {
return a * b;
}
public int add(int a, int b) {
return a + b;
}
public int substract(int a, int b) {
return a - b;
}
}
ExampleSessionBeanLocal source:
package example;
import javax.ejb.Local;
@Local
public interface ExampleSessionBeanLocal {
public int multiply(int a, int b);
public int add(int a, int b);
public int substract(int a, int b);
}
ExampleSessionBeanRemote source:
package example;
import javax.ejb.Remote;
@Remote
public interface ExampleSessionBeanRemote {
public int multiply(int a, int b);
public int add(int a, int b);
public int substract(int a, int b);
}
Step 7: Export project as EJB jar
To compile the EJB-jar file, we select the project and do a right-click. Now select Export and then EJB JAR file. Now fill in the next screens:
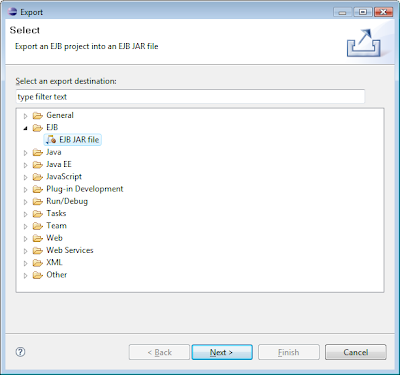
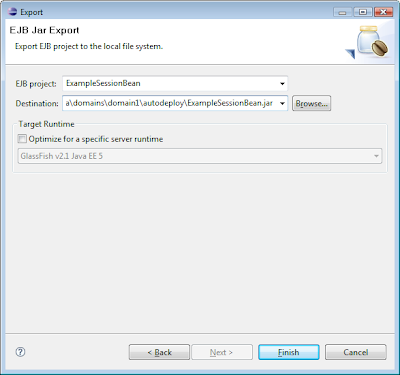
Step 8: Deploy the EJB-jar
Now drag and drop the exported EJB-jar file to the autodeploy directory of Glassfish. The bean is now ready for use.
Step 9: Create remote test client
The step described next is optional. In this step we create a test client, which is run within Eclipse to test the remote EJB interface we created.
import javax.naming.InitialContext;
import example.ExampleSessionBeanRemote;
public class Main {
public void runTest() throws Exception {
InitialContext ctx = new InitialContext();
ExampleSessionBeanRemote bean =
(ExampleSessionBeanRemote) ctx
.lookup("ejb/ExampleSessionBean");
int result = bean.multiply(3, 4);
System.out.println(result);
}
public static void main(String[] args) {
Main cli = new Main();
try {
cli.runTest();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Compile and run within Eclipse to view the result in the Console tab.
good kick start
ReplyDeletehow do i create a test client to deploy my project
Deletegood one
ReplyDeletejavax.naming.NoInitialContextException: Need to specify class name in environment or system property, or as an applet parameter, or in an application resource file: java.naming.factory.initial
ReplyDeleteat javax.naming.spi.NamingManager.getInitialContext(NamingManager.java:662)
at javax.naming.InitialContext.getDefaultInitCtx(InitialContext.java:307)
at javax.naming.InitialContext.getURLOrDefaultInitCtx(InitialContext.java:344)
at javax.naming.InitialContext.lookup(InitialContext.java:411)
at example.Main.runTest(Main.java:10)
at example.Main.main(Main.java:18)
You can use this utility class to create your InitialContext:
Deleteimport java.util.Properties;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
public class Utility {
/*
* location of JBoss JNDI Service provider the client will use. It should be
* URL string.
*/
private static final String PROVIDER_URL = "jnp://localhost:1099";
/*
* specifying the list of package prefixes to use when loading in URL
* context factories. colon separated
*/
private static final String JNP_INTERFACES = "org.jboss.naming:org.jnp.interfaces";
/*
* Factory that creates initial context objects. fully qualified class name.
*/
private static final String INITIAL_CONTEXT_FACTORY = "org.jnp.interfaces.NamingContextFactory";
private static Context initialContext;
public static Context getInitialContext() throws NamingException {
if (initialContext == null) {
// Properties extends HashTable
Properties prop = new Properties();
prop.put(Context.INITIAL_CONTEXT_FACTORY, INITIAL_CONTEXT_FACTORY);
prop.put(Context.URL_PKG_PREFIXES, JNP_INTERFACES);
prop.put(Context.PROVIDER_URL, PROVIDER_URL);
initialContext = new InitialContext(prop);
}
return initialContext;
}
}
This will work with JBoss instead of the GlassFish
Deletehelpful :)
ReplyDeletethanks